A cheat sheet for Terraform CLI
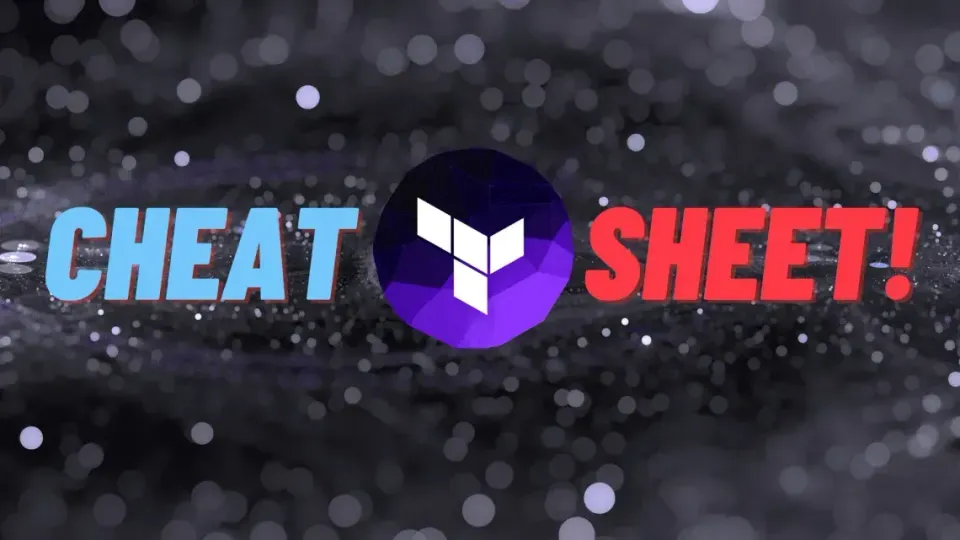
This is a cheat sheet for Terraform.
Terraform CLI Bash
Setup tab auto-completion, requires logging back in
terraform -install-autocomplete
Format and Validate Terraform Code
Format code
terraform fmt
Validate code for synthax errors
terraform validate
Validate code without checking backend validation
terraform validate -backend=false
Terraform Init
Initialize Terraform in current directory, pull down providers
terraform init
Initialize Terraform in current directory, do not pull down providers
terraform init -get-plugins=false
Initialize Terraform in current directory, do not verify Hashicorp signature
terraform init -verify-plugins=false
Plan, Apply and Utilities
Apply changes without being prompted to enter “yes”
terraform apply --auto-approve
Destroy infrastructure without being prompted to enter “yes”
terraform destroy --auto-approve
Output the plan to a file : plan.out
terraform apply plan.out
Plan a destroy
terraform plan -destroy
Apply on a target resource
terraform apply -target=aws_instance.my_ec2
Variables into command
terraform apply -var my_region_variable=eu-central-1
Lock : the state file so it can’t be modified by any other Terraform apply or modification action (possible only where backend allows locking)
terraform apply -lock=true
Refresh : Do not reconcile state file with real-world resources(helpful with large complex deployments for saving deployment time)
terraform apply refresh=false
Refresh : Reconcile the state in Terraform state file with real-world resources
terraform refresh
Parallelism : Number of simultaneous resource operations
terraform apply --parallelism=5
Providers : get informations about the current providers
terraform providers
Terraform Workspaces
Create a new workspace
terraform workspace new mynewworkspace
Switch to another workspace
terraform workspace select anotherworkspace
List all workspaces
terraform workspace list
Terraform State
Show details stored in Terraform state for the resource
terraform state show aws_instance.my_ec2
Pull terraform state to a file
terraform state pull > terraform.tfstate
Move a resource tracked via state to different module
terraform state mv aws_iam_role.my_ssm_role module.custom_module
Replace an existing provider with another
terraform state replace-provider hashicorp/aws registry.custom.com/aws
List out all the resources tracked via the current state file
terraform state list
Remove a resource from the state, it doesn’t delete the resource
terraform state rm aws_instance.myinstace
Terraform Import
Import EC2 instance
terraform import aws_instance.new_ec2_instance i-abcd1234
Import EC2 instance with a count
terraform import 'aws_instance.new_ec2_instance[0]' i-abcd1234
Terraform Outputs
List all outputs as stated in code
terraform output
List out a specific declared output
terraform output instance_public_ip
List all outputs in JSON format
terraform output -json
Terraform Taint
Taint resource to be recreated on next apply
terraform taint aws_instance.my_ec2
Remove taint from a taint resource
terraform untaint aws_instance.my_ec2
Terraform Console
echo an expression into terraform console and see its expected result as output
echo 'join(",",["foo","bar"])' | terraform console
Terraform console also has an interactive CLI just enter “terraform console”
echo '1 + 5' | terraform console
Display the Public IP against the “my_ec2” Terraform resource as seen in the Terraform state file
echo "aws_instance.my_ec2.public_ip" | terraform console
Terraform Graph
Produce a PNG diagrams showing relationship and dependencies
terraform graph | dot -Tpng > graph.png
Terraform Cloud
Obtain and save API token for Terraform cloud
terraform login
Log out of Terraform Cloud, defaults to hostname app.terraform.io
terraform logout
Terraform Miscelleneous
Show version
terraform version
Download and update modules in the “root” module.
terraform get -update=true